I’m trying to crop a part of the image randomly and it seems to me the RandomResizedCrop class fits the bill perfectly. It’s just that for some reason, across different runs, the transformation always picks the exact same spot from the original image and that is the middle section. I made sure that I’m not setting any seeds for the random packages and I don’t know what I’m doing wrong.
This is the code I have:
from torchvision.io import read_image
from torchvision.transforms import v2
import matplotlib.pyplot as plt
img = read_image("./some_image.png")
t = v2.Compose([
lambda x: x / 255.0,
v2.RandomResizedCrop(size=64),
])
v = t(img)
plt.imshow(img.permute(1, 2, 0))
plt.show()
plt.imshow(v.permute(1, 2, 0))
plt.show()
I cannot reproduce the issue and get different crops when closing the terminal and rerunning the script.
Could you please test it with this image? It’s one sample from IAM Handwritten text dataset:
Maybe it has something to do with the image size!? For me it always returns:
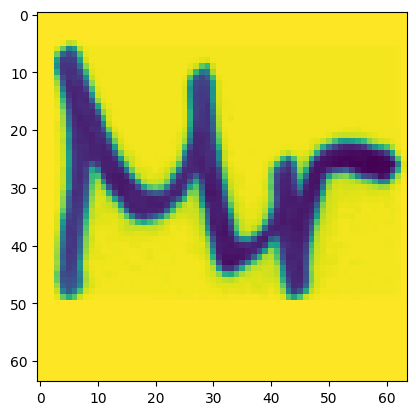
By always I mean when I rerun the same Jupyer notebook cell. And I’m running the same exact code above (just changing the file path).
For the record, I just tested the code with the sample image in a Google Colab and I can reproduce the problem. I created two cells with the same code and both of them output the same crop of the image (depicted above). Either my expectations are not aligned or this is a bug. It would be great if someone could please confirm one or the other.
I can reproduce the central crop using your flat image and it seems this random sampling fails giving e.g.:
failed with: w: 114, h: 130, width: 1661, height: 89
failed with: w: 341, h: 349, width: 1661, height: 89
failed with: w: 190, h: 221, width: 1661, height: 89
failed with: w: 356, h: 282, width: 1661, height: 89
failed with: w: 345, h: 342, width: 1661, height: 89
failed with: w: 362, h: 336, width: 1661, height: 89
failed with: w: 124, h: 112, width: 1661, height: 89
failed with: w: 358, h: 291, width: 1661, height: 89
failed with: w: 346, h: 332, width: 1661, height: 89
failed with: w: 410, h: 332, width: 1661, height: 89
after adding some debug print
statements.
In cast the sampled h
is too large. You might thus need to reduce the scale
.